Flutter Best Practices — Part 6
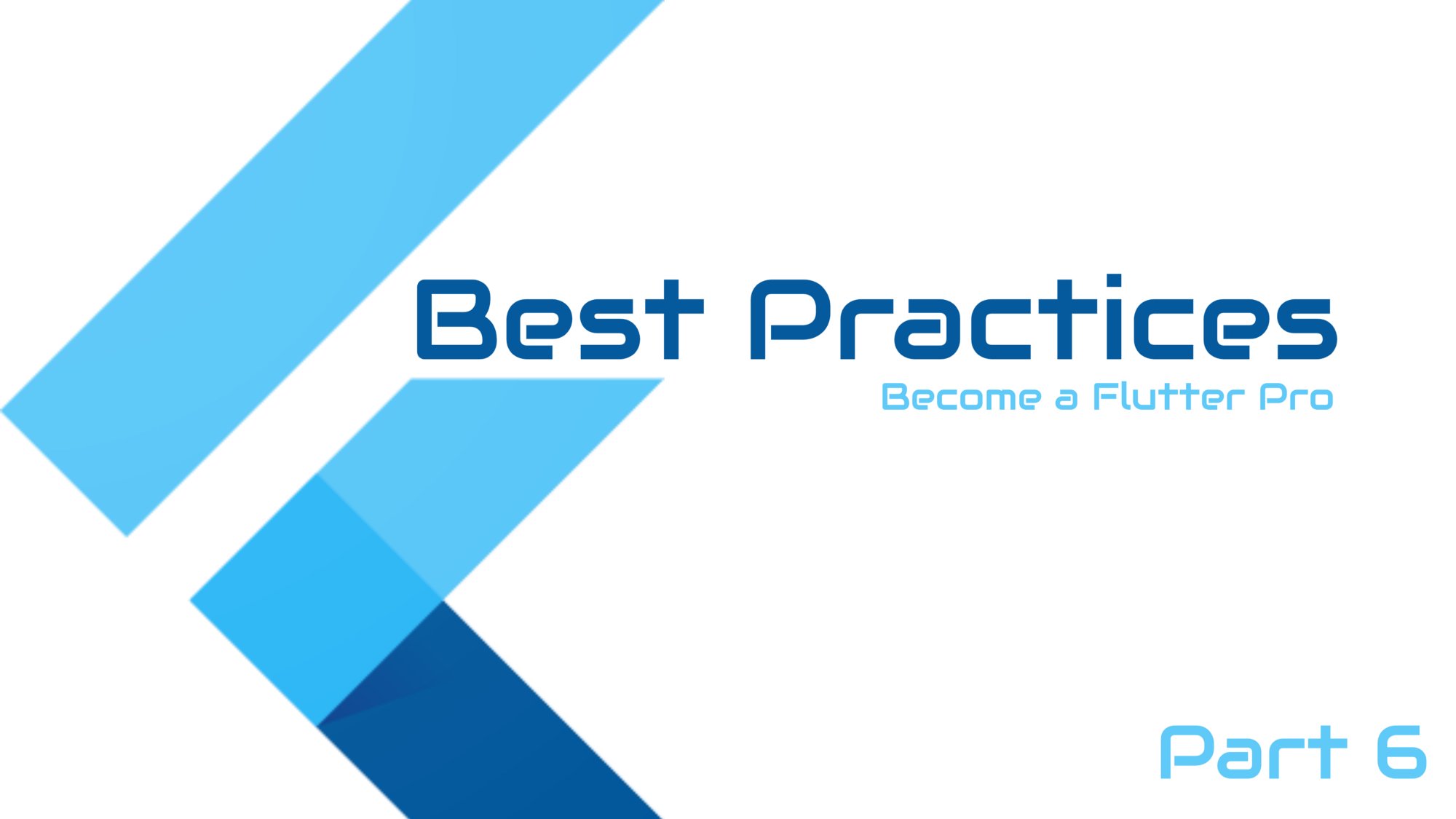
Proper naming for Magic Numbers
// Do not SvgPicture.asset( Images.frameWhite, height: 13.0, width: 13.0, ); // Do final _frameIconSize = 13.0; SvgPicture.asset( Images.frameWhite, height: _frameIconSize, width: _frameIconSize, );
Understanding the concept of constraints in Flutter
There is a thumb rule of a Flutter layout that every Flutter app developer needs to know: constraints go down, sizes go up, and the parent sets the position.
Examples might be pretty bigger to mention everything here.
Widgets choice matters
Poor Choice of widget might lead to bigger trees which causes bigger build time causing performance issues.
Use packages only when necessary
Packages can be a good choice when you want to speed up your building process and pub has a ton of them. Nonetheless, they can have significant effects on your app performances when they are used without prior study.
Linting
When you create a project with the latest versions of Flutter, the analysis_options.yml file is created by default with the official lint rules.
Visit https://flutter.dev/docs/perf/rendering/best-practices for a complete reference about the two first points, and more other good practices.
Do something after the build completes can improve performance
@override void initState() { super.initState(); WidgetsBinding.instance.addPostFrameCallback((timeStamp) { doSomething(); }); }
Use SafeArea
SafeArea widget insets child by removing padding required to OS controls like status bar, bottom navigations buttons like back button etc.
@override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(), body: SafeArea( child: YourWidget(), }, }; }
Use keys to improve Flutter performance
This provides better performance by using the flutter of keys to better identify widgets.
// FROM return value ? const SizedBox() : const Placeholder(), // TO return value ? const SizedBox(key: ValueKey('SizedBox')) : const Placeholder(key: ValueKey('Placeholder')), ---------------------------------------------- // FROM final inner = SizedBox(); return value ? SizedBox(child: inner) : inner, // TO final global = GlobalKey(); final inner = SizedBox(key: global); return value ? SizedBox(child: inner) : inner,
Analyze & Decrease Application Size
At the time of development, it is easy to use multiple packages, codes, and widgets in an application. But sometimes, it requires high memory to store all of this data, which also decreases the application’s performance.
Flutter’s development tool provides an advantage of reducing the application size. With the help of Gradle, you can reduce the Flutter application size to optimize Flutter performance.
Using the packaging system introduced by Google, you can create bundles of Android applications. App Bundles are beneficial in many ways. One of the main features of the app bundle is that it allows you to download original code from the Google Play Store. Google Play Store provides applications that are compatible with the device and supports the platform’s architecture.
flutter build appbundle
iOS:
Optimize memory when using image ListView
ListView.builder( ... addAutomaticKeepAlives: false (true by default) addRepaintBoundaries: false (true by default) );
Display your images and icons precisely
Pre-cache the images for better performance.
precacheImage(AssetImage(imagePath), context);
Use SKSL Warmup
flutter run --profile --cache-sksl --purge-persistent-cache flutter build apk --cache-sksl --purge-persistent-cache
If an application has clean animations on the first run, and then becomes smooth for the same animations, it is likely due to delays in shader compilation.
Don’t use references in List Maps
Don’t
List a = [1,2,3,4]; List b; b = a; a.remove(1); print(a); // [2,3,4] print(b); // [2,3,4]
For this reason, whenever you try to call any method of list a, list b is automatically called.
For example a.remove (some) ; will also remove the item from list b;
Do
List a = [1,2,3,4]; List b; b = jsonDecode(jsonEncode(a)); a.remove(1); print(a); // [2,3,4] print(b); // [1,2,3,4]
Avoid Absolute Positioning At All Costs
Absolute positioning is one of the worst things you can do when building apps. When coding for just one specific device, it can seem like absolute positioning is not that bad. However, when you’re building an app that’s meant to be released onto various different devices and operating systems — absolute positioning can really mess up the interface. Use values that adapt to the sizes of screens instead of absolute positioning whenever possible
Quick Tips:
Official Documentation
https://docs.flutter.dev/perf/best-practices
Using DevTools for Performance testing:
https://docs.flutter.dev/development/tools/devtools/performance
https://api.flutter.dev/flutter/widgets/StatefulWidget-class.html#performance-considerations
Opacity class – widgets library – Dart API
Previous Episodes
Flutter — Best Practices — Part 1Flutter Best Practices — Part 2Flutter Best Practices — Part 3Flutter Best Practices — Part 4Flutter Best Practices — Part 5
Hope you enjoy this article. Please let me know in the comments.
Please clap if you found it useful.
Thanks for reading.
Leave a Reply